C Program to Check Whether a Character is Vowel or Consonant
In this example, if...else statement is used to check whether an alphabet entered by the user is a vowel or a constant.
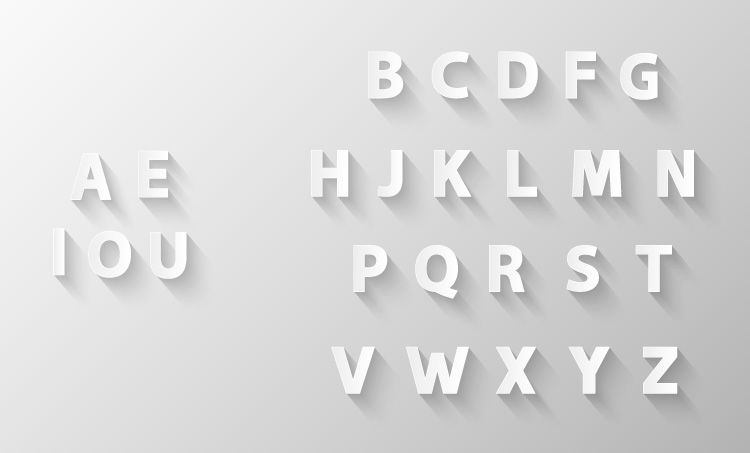
To understand this example, you should have the knowledge of following C programming topics:
The five alphabets A, E, I, O and U are called vowels. All other alphabets except these 5 vowel letters are called consonants.
This program assumes that the user will always enter an alphabet character.
The isLowerCaseVowel evaluates to 1 (true) if c is a lowercase vowel and 0 (false) for any other character.
Similarly, isUpperCaseVowel evaluates to 1(true) if c is an uppercase vowel and 0 (false) for any other character.
If both isLowercaseVowel and isUppercaseVowel is equal to 0, the test expression evaluates to 0 (false) and the entered character is a consonant.
However, if either isLowercaseVowel or isUppercaseVowel is 1 (true), the test expression evaluates to 1 (true) and the entered character is a vowel.
The program above assumes that the user always enters an alphabet. If the user enters any other character other than an alphabet, the program will not work as intended.
This program assumes that the user will always enter an alphabet character.
Example #1: Program to Check Vowel or consonant
#include <stdio.h>
int main()
{
char c;
int isLowercaseVowel, isUppercaseVowel;
printf("Enter an alphabet: ");
scanf("%c",&c);
// evaluates to 1 (true) if c is a lowercase vowel
isLowercaseVowel = (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u');
// evaluates to 1 (true) if c is an uppercase vowel
isUppercaseVowel = (c == 'A' || c == 'E' || c == 'I' || c == 'O' || c == 'U');
// evaluates to 1 (true) if either isLowercaseVowel or isUppercaseVowel is true
if (isLowercaseVowel || isUppercaseVowel)
printf("%c is a vowel.", c);
else
printf("%c is a consonant.", c);
return 0;
}
OutputEnter an alphabet: G G is a consonant.The character entered by the user is stored in variable c.
The isLowerCaseVowel evaluates to 1 (true) if c is a lowercase vowel and 0 (false) for any other character.
If both isLowercaseVowel and isUppercaseVowel is equal to 0, the test expression evaluates to 0 (false) and the entered character is a consonant.
However, if either isLowercaseVowel or isUppercaseVowel is 1 (true), the test expression evaluates to 1 (true) and the entered character is a vowel.
The program above assumes that the user always enters an alphabet. If the user enters any other character other than an alphabet, the program will not work as intended.
Example #2: Program to Check Vowel or consonant
The program below asks the user to enter a character until the user enters an alphabet. Then, the program checks whether it is a vowel or a consonant.#include <stdio.h>
#include <ctype.h>
int main()
{
char c;
int isLowercaseVowel, isUppercaseVowel;
do {
printf("Enter an alphabet: ");
scanf(" %c", &c);
}
// isalpha() returns 0 if the passed character is not an alphabet
while (!isalpha(c));
// evaluates to 1 (true) if c is a lowercase vowel
isLowercaseVowel = (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u');
// evaluates to 1 (true) if c is an uppercase vowel
isUppercaseVowel = (c == 'A' || c == 'E' || c == 'I' || c == 'O' || c == 'U');
// evaluates to 1 (true) if either isLowercaseVowel or isUppercaseVowel is true
if (isLowercaseVowel || isUppercaseVowel)
printf("%c is a vowel.", c);
else
printf("%c is a consonant.", c);
return 0;
}
Comments
Post a Comment